Introduction
JAX has arange emerged as a powerful tool for numerical computing and machine learning, offering a unique combination of high-performance computing with the familiar syntax of NumPy. As a library that accelerates numerical calculations with automatic differentiation and just-in-time (JIT) compilation, JAX is essential for anyone working with data-intensive tasks.
One key feature of JAX is its array manipulation capabilities. jax.arange is a fundamental function that allows users to create arrays efficiently, similar to its NumPy counterpart. Additionally, JAX introduces advanced loop control mechanisms like loop carry, which enable efficient handling of iterative computations.
This guide delves deep into the combination of jax.arange with loop carry to optimize performance. Understanding how to effectively use these tools can significantly improve the efficiency and readability of JAX-based code.
Understanding jax.arange
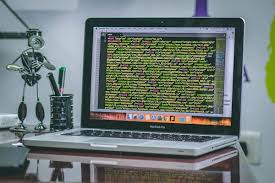
What is jax.arange?
jax.arange is a function that generates evenly spaced values over a specified range. It is analogous to NumPy’s arange, but optimized for JAX’s computation model. The syntax is straightforward:
import jax.numpy as jnp
arr = jnp.arange(start, stop, step)
Comparison with NumPy’s arange
While the functionality is similar, JAX’s arange benefits from automatic differentiation and GPU/TPU support. This makes it ideal for deep learning and scientific computing tasks.
Syntax and Parameters
- start (optional): The starting value of the sequence (default is 0).
- stop: The end value of the sequence (exclusive).
- step (optional): The interval between consecutive values (default is 1).
Practical Examples
# Create an array from 0 to 9
arr = jnp.arange(10)
# Create an array from 1 to 10 with step size of 2
arr = jnp.arange(1, 11, 2)
These arrays are commonly used for indexing, slicing, and iteration in JAX programs.
Introduction to Loop Carry in JAX
What is Loop Carry?
Loop carry is a mechanism that allows values to be passed from one iteration of a loop to the next. In JAX, this is essential for operations that require iterative computation without losing state.
Role in Iterative Computations
Loop carry is crucial in dynamic programming, optimization problems, and machine learning algorithms like gradient descent. By maintaining state across iterations, it avoids reinitialization and enhances performance.
Common Scenarios for Use
- Gradient descent optimization
- Cumulative summation
- Matrix multiplication loops
Benefits of Loop Carry
- Memory Efficiency: By updating state in-place, loop carry reduces memory overhead.
- Speed: It allows better utilization of JAX’s just-in-time compilation.
- Code Clarity: It simplifies complex iterative computations.
A basic loop carry example in JAX uses the lax.scan function:
from jax import lax
def loop_body(carry, x):
carry = carry + x
return carry, carry
result = lax.scan(loop_body, 0, jnp.arange(5))
Using jax.arange with Loop Carry: Step-by-Step Guide
Combining jax.arange with Loop Carry
To harness the full power of JAX, combining jax.arange with loop carry is essential. This combination is often used to iterate through arrays while maintaining state efficiently.
Step-by-Step Example
Consider an example where we compute the cumulative sum of an array:
import jax.numpy as jnp
from jax import lax
# Array initialization using jax.arange
arr = jnp.arange(1, 6)
# Function to perform cumulative summation
def cumulative_sum(carry, x):
carry = carry + x
return carry, carry
# Using lax.scan to apply loop carry
result, _ = lax.scan(cumulative_sum, 0, arr)
print(result)
This code calculates the cumulative sum [1, 3, 6, 10, 15] efficiently by leveraging loop carry.
Handling Edge Cases
- Empty Arrays: Ensure proper handling to avoid errors.
- Non-integer Steps: Use floating-point arrays carefully to avoid precision issues.
Performance Considerations
JAX’s just-in-time (JIT) compilation optimizes the loop carry process, making it faster than traditional loops in NumPy.
Best Practices and Optimization Tips
Choosing Appropriate Array Sizes
Selecting the right array size is critical for performance. Large arrays may require memory management techniques.
Static vs. Dynamic Arrays
Use static arrays when possible to take advantage of JIT compilation. Dynamic arrays may lead to performance penalties.
Memory Management
Efficient memory usage is key in JAX. Avoid creating unnecessary arrays and use in-place updates whenever possible.
Debugging Tips
- Use JAX’s print functionality for debugging loop carry values.
- Use smaller arrays for testing before scaling up.
Real-World Examples
In deep learning, jax.arange and loop carry are often used for sequential data processing, such as RNNs or time-series forecasting.
Conclusion
Mastering jax.arange and loop carry is essential for optimizing JAX programs. These tools enable efficient array manipulation and iterative computations, making them invaluable for machine learning and scientific computing tasks. By practicing with real-world examples, you can harness the full potential of JAX for performance and clarity.